# @ohos.promptAction (弹窗)
创建并显示文本提示框、对话框和操作菜单。
> **说明:**
>
> 本模块首批接口从API version 9开始支持。后续版本的新增接口,采用上角标单独标记接口的起始版本。
>
> 该模块不支持在[UIAbility](../apis-ability-kit/js-apis-app-ability-uiAbility.md)的文件声明处使用,即不能在UIAbility的生命周期中调用,需要在创建组件实例后使用。
>
> 本模块功能依赖UI的执行上下文,不可在UI上下文不明确的地方使用,参见[UIContext](js-apis-arkui-UIContext.md#uicontext)说明。建议在除[ServiceExtension](../../application-models/serviceextensionability.md)等无UI界面的场景外,均使用UIContext中的弹窗方法。
>
> 从API version 10开始,可以通过使用[UIContext](js-apis-arkui-UIContext.md#uicontext)中的[getPromptAction](js-apis-arkui-UIContext.md#getpromptaction)方法获取当前UI上下文关联的[PromptAction](js-apis-arkui-UIContext.md#promptaction)对象。
## 导入模块
```ts
import { promptAction } from '@kit.ArkUI';
```
## promptAction.showToast
showToast(options: ShowToastOptions): void
创建并显示文本提示框。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
**参数:**
| 参数名 | 类型 | 必填 | 说明 |
| ------- | ------------------------------------- | ---- | ------- |
| options | [ShowToastOptions](#showtoastoptions) | 是 | 文本弹窗选项。 |
**错误码:**
以下错误码的详细介绍请参见[通用错误码](../errorcode-universal.md)和[ohos.promptAction(弹窗)](errorcode-promptAction.md)错误码。
| 错误码ID | 错误信息 |
| --------- | ------- |
| 401 | Parameter error. Possible causes: 1. Mandatory parameters are left unspecified; 2.Incorrect parameters types; 3. Parameter verification failed. |
| 100001 | Internal error. |
**示例:**
```ts
import { promptAction } from '@kit.ArkUI'
import { BusinessError } from '@kit.BasicServicesKit';
@Entry
@Component
struct toastExample {
build() {
Column() {
Button('Show toast').fontSize(20)
.onClick(() => {
try {
promptAction.showToast({
message: 'Hello World',
duration: 2000
});
} catch (error) {
let message = (error as BusinessError).message
let code = (error as BusinessError).code
console.error(`showToast args error code is ${code}, message is ${message}`);
};
})
}.height('100%').width('100%').justifyContent(FlexAlign.Center)
}
}
```
API version 11及之前Toast样式。
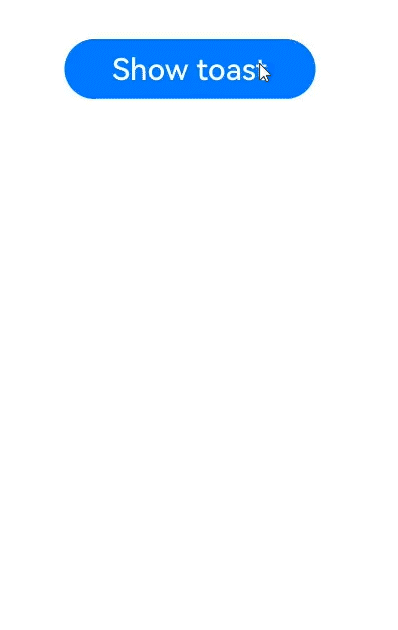
API version 12及之后Toast样式。
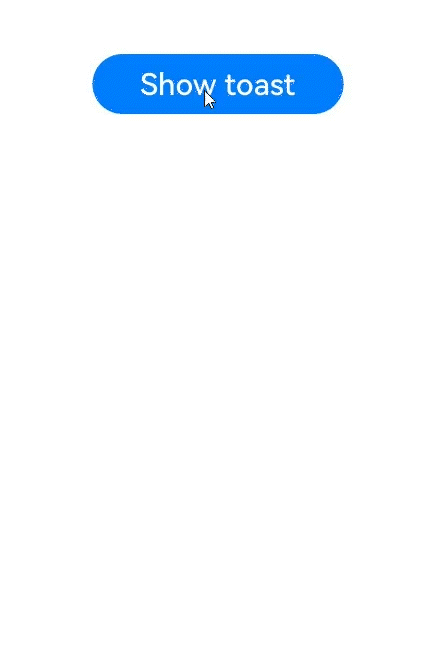
## promptAction.showDialog
showDialog(options: ShowDialogOptions): Promise<ShowDialogSuccessResponse>
创建并显示对话框,对话框响应后异步返回结果。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
**参数:**
| 参数名 | 类型 | 必填 | 说明 |
| ------- | --------------------------------------- | ---- | ------ |
| options | [ShowDialogOptions](#showdialogoptions) | 是 | 对话框选项。 |
**返回值:**
| 类型 | 说明 |
| ---------------------------------------- | -------- |
| Promise<[ShowDialogSuccessResponse](#showdialogsuccessresponse)> | 对话框响应结果。 |
**错误码:**
以下错误码的详细介绍请参见[通用错误码](../errorcode-universal.md)和[ohos.promptAction(弹窗)](errorcode-promptAction.md)错误码。
| 错误码ID | 错误信息 |
| --------- | ------- |
| 401 | Parameter error. Possible causes: 1. Mandatory parameters are left unspecified; 2.Incorrect parameters types; 3. Parameter verification failed. |
| 100001 | Internal error. |
**示例:**
```ts
import { promptAction } from '@kit.ArkUI'
import { BusinessError } from '@kit.BasicServicesKit';
try {
promptAction.showDialog({
title: 'Title Info',
message: 'Message Info',
buttons: [
{
text: 'button1',
color: '#000000'
},
{
text: 'button2',
color: '#000000'
}
],
})
.then(data => {
console.info('showDialog success, click button: ' + data.index);
})
.catch((err:Error) => {
console.info('showDialog error: ' + err);
})
} catch (error) {
let message = (error as BusinessError).message
let code = (error as BusinessError).code
console.error(`showDialog args error code is ${code}, message is ${message}`);
};
```

## promptAction.showDialog
showDialog(options: ShowDialogOptions, callback: AsyncCallback<ShowDialogSuccessResponse>):void
创建并显示对话框,对话框响应结果异步返回。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
**参数:**
| 参数名 | 类型 | 必填 | 说明 |
| -------- | ---------------------------------------- | ---- | ------------ |
| options | [ShowDialogOptions](#showdialogoptions) | 是 | 页面显示对话框信息描述。 |
| callback | AsyncCallback<[ShowDialogSuccessResponse](#showdialogsuccessresponse)> | 是 | 对话框响应结果回调。 |
**错误码:**
以下错误码的详细介绍请参见[通用错误码](../errorcode-universal.md)和[ohos.promptAction(弹窗)](errorcode-promptAction.md)错误码。
| 错误码ID | 错误信息 |
| --------- | ------- |
| 401 | Parameter error. Possible causes: 1. Mandatory parameters are left unspecified; 2.Incorrect parameters types; 3. Parameter verification failed. |
| 100001 | Internal error. |
**示例:**
```ts
import { promptAction } from '@kit.ArkUI';
import { BusinessError } from '@kit.BasicServicesKit';
try {
promptAction.showDialog({
title: 'showDialog Title Info',
message: 'Message Info',
buttons: [
{
text: 'button1',
color: '#000000'
},
{
text: 'button2',
color: '#000000'
}
]
}, (err, data) => {
if (err) {
console.info('showDialog err: ' + err);
return;
}
console.info('showDialog success callback, click button: ' + data.index);
});
} catch (error) {
let message = (error as BusinessError).message
let code = (error as BusinessError).code
console.error(`showDialog args error code is ${code}, message is ${message}`);
};
```

当弹窗的showInSubWindow属性为true时,弹窗可显示在窗口外。
```ts
import { promptAction } from '@kit.ArkUI';
import { BusinessError } from '@kit.BasicServicesKit';
try {
promptAction.showDialog({
title: 'showDialog Title Info',
message: 'Message Info',
isModal: true,
showInSubWindow: true,
buttons: [
{
text: 'button1',
color: '#000000'
},
{
text: 'button2',
color: '#000000'
}
]
}, (err, data) => {
if (err) {
console.info('showDialog err: ' + err);
return;
}
console.info('showDialog success callback, click button: ' + data.index);
});
} catch (error) {
let message = (error as BusinessError).message
let code = (error as BusinessError).code
console.error(`showDialog args error code is ${code}, message is ${message}`);
};
```

## promptAction.showActionMenu
showActionMenu(options: ActionMenuOptions, callback: AsyncCallback<ActionMenuSuccessResponse>):void
创建并显示操作菜单,菜单响应结果异步返回。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full。
**参数:**
| 参数名 | 类型 | 必填 | 说明 |
| -------- | ---------------------------------------- | ---- | --------- |
| options | [ActionMenuOptions](#actionmenuoptions) | 是 | 操作菜单选项。 |
| callback | AsyncCallback<[ActionMenuSuccessResponse](#actionmenusuccessresponse)> | 是 | 菜单响应结果回调。 |
**错误码:**
以下错误码的详细介绍请参见[通用错误码](../errorcode-universal.md)和[ohos.promptAction(弹窗)](errorcode-promptAction.md)错误码。
| 错误码ID | 错误信息 |
| --------- | ------- |
| 401 | Parameter error. Possible causes: 1. Mandatory parameters are left unspecified; 2.Incorrect parameters types; 3. Parameter verification failed. |
| 100001 | Internal error. |
**示例:**
```ts
import { promptAction } from '@kit.ArkUI';
import { BusinessError } from '@kit.BasicServicesKit';
try {
promptAction.showActionMenu({
title: 'Title Info',
buttons: [
{
text: 'item1',
color: '#666666'
},
{
text: 'item2',
color: '#000000'
},
]
}, (err, data) => {
if (err) {
console.info('showActionMenu err: ' + err);
return;
}
console.info('showActionMenu success callback, click button: ' + data.index);
})
} catch (error) {
let message = (error as BusinessError).message
let code = (error as BusinessError).code
console.error(`showActionMenu args error code is ${code}, message is ${message}`);
};
```

## promptAction.showActionMenu
showActionMenu(options: ActionMenuOptions): Promise<ActionMenuSuccessResponse>
创建并显示操作菜单,菜单响应后异步返回结果。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
**参数:**
| 参数名 | 类型 | 必填 | 说明 |
| ------- | --------------------------------------- | ---- | ------- |
| options | [ActionMenuOptions](#actionmenuoptions) | 是 | 操作菜单选项。 |
**返回值:**
| 类型 | 说明 |
| ---------------------------------------- | ------- |
| Promise<[ActionMenuSuccessResponse](#actionmenusuccessresponse)> | 菜单响应结果。 |
**错误码:**
以下错误码的详细介绍请参见[通用错误码](../errorcode-universal.md)和[ohos.promptAction(弹窗)](errorcode-promptAction.md)错误码。
| 错误码ID | 错误信息 |
| --------- | ------- |
| 401 | Parameter error. Possible causes: 1. Mandatory parameters are left unspecified; 2.Incorrect parameters types; 3. Parameter verification failed. |
| 100001 | Internal error. |
**示例:**
```ts
import { promptAction } from '@kit.ArkUI';
import { BusinessError } from '@kit.BasicServicesKit';
try {
promptAction.showActionMenu({
title: 'showActionMenu Title Info',
buttons: [
{
text: 'item1',
color: '#666666'
},
{
text: 'item2',
color: '#000000'
},
]
})
.then(data => {
console.info('showActionMenu success, click button: ' + data.index);
})
.catch((err:Error) => {
console.info('showActionMenu error: ' + err);
})
} catch (error) {
let message = (error as BusinessError).message
let code = (error as BusinessError).code
console.error(`showActionMenu args error code is ${code}, message is ${message}`);
};
```

## promptAction.openCustomDialog11+
openCustomDialog(options: CustomDialogOptions): Promise<number>
打开自定义弹窗。
不支持在ServiceExtension中使用。
暂不支持isModal = true与showInSubWindow = true同时使用。
弹窗宽度在设备竖屏时默认为 所在窗口宽度 - 左右margin(16vp,设备为2in1时为40vp),最大默认宽度为400vp。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
**参数:**
| 参数名 | 类型 | 必填 | 说明 |
| ------- | --------------------------------------------- | ---- | ------------------ |
| options | [CustomDialogOptions](#customdialogoptions11) | 是 | 自定义弹窗的内容。 |
**返回值:**
| 类型 | 说明 |
| --------------------- | ------------------------------------- |
| Promise<number> | 返回供closeCustomDialog使用的对话框id。 |
**错误码:**
以下错误码的详细介绍请参见[通用错误码](../errorcode-universal.md)和[ohos.promptAction(弹窗)](errorcode-promptAction.md)错误码。
| 错误码ID | 错误信息 |
| -------- | ---------------------------------- |
| 401 | Parameter error. Possible causes: 1. Mandatory parameters are left unspecified; 2.Incorrect parameters types; 3. Parameter verification failed. |
| 100001 | Internal error. |
**示例:**
```ts
import { promptAction } from '@kit.ArkUI'
import { BusinessError } from '@kit.BasicServicesKit'
@Entry
@Component
struct Index {
private customDialogComponentId: number = 0
@Builder
customDialogComponent() {
Column() {
Text('弹窗').fontSize(30)
Row({ space: 50 }) {
Button("确认").onClick(() => {
try {
promptAction.closeCustomDialog(this.customDialogComponentId)
} catch (error) {
let message = (error as BusinessError).message;
let code = (error as BusinessError).code;
console.error(`closeCustomDialog error code is ${code}, message is ${message}`);
}
})
Button("取消").onClick(() => {
try {
promptAction.closeCustomDialog(this.customDialogComponentId)
} catch (error) {
let message = (error as BusinessError).message;
let code = (error as BusinessError).code;
console.error(`closeCustomDialog error code is ${code}, message is ${message}`);
}
})
}
}.height(200).padding(5).justifyContent(FlexAlign.SpaceBetween)
}
build() {
Row() {
Column({ space: 20 }) {
Text('组件内弹窗')
.fontSize(30)
.onClick(() => {
promptAction.openCustomDialog({
builder: () => {
this.customDialogComponent()
},
onWillDismiss: (dismissDialogAction: DismissDialogAction) => {
console.info("reason" + JSON.stringify(dismissDialogAction.reason))
console.log("dialog onWillDismiss")
if (dismissDialogAction.reason == DismissReason.PRESS_BACK) {
dismissDialogAction.dismiss()
}
if (dismissDialogAction.reason == DismissReason.TOUCH_OUTSIDE) {
dismissDialogAction.dismiss()
}
}
}).then((dialogId: number) => {
this.customDialogComponentId = dialogId
})
.catch((error: BusinessError) => {
console.error(`openCustomDialog error code is ${error.code}, message is ${error.message}`)
})
})
}
.width('100%')
}
.height('100%')
}
}
```
该示例定义了弹窗样式,如宽度、高度、背景色、阴影等等。
```ts
import { promptAction } from '@kit.ArkUI'
let customDialogId: number = 0
@Builder
function customDialogBuilder() {
Column() {
Text('Custom dialog Message').fontSize(10)
Row() {
Button("确认").onClick(() => {
promptAction.closeCustomDialog(customDialogId)
})
Blank().width(50)
Button("取消").onClick(() => {
promptAction.closeCustomDialog(customDialogId)
})
}
}
}
@Entry
@Component
struct Index {
@State message: string = 'Hello World'
@Builder
customDialogComponent() {
customDialogBuilder()
}
build() {
Row() {
Column() {
Text(this.message)
.fontSize(50)
.fontWeight(FontWeight.Bold)
.onClick(() => {
promptAction.openCustomDialog({
builder: () => {
this.customDialogComponent()
},
keyboardAvoidMode: KeyboardAvoidMode.NONE,
showInSubWindow: false,
offset: { dx: 5, dy: 5 },
backgroundColor: 0xd9ffffff,
cornerRadius: 20,
width: '80%',
height: 200,
borderWidth: 1,
borderStyle: BorderStyle.Dashed, //使用borderStyle属性,需要和borderWidth属性一起使用
borderColor: Color.Blue, //使用borderColor属性,需要和borderWidth属性一起使用
shadow: ({
radius: 20,
color: Color.Grey,
offsetX: 50,
offsetY: 0
}),
}).then((dialogId: number) => {
customDialogId = dialogId
})
})
}
.width('100%')
}
.height('100%')
}
}
```
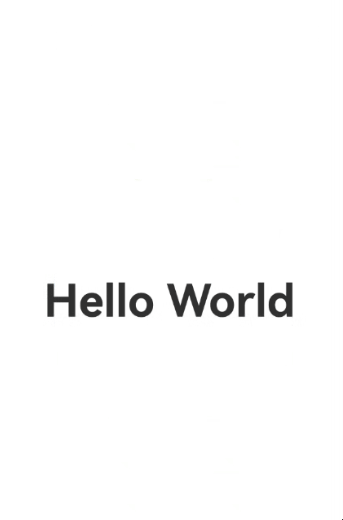
## promptAction.closeCustomDialog11+
closeCustomDialog(dialogId: number): void
关闭自定义弹窗。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
**参数:**
| 参数名 | 类型 | 必填 | 说明 |
| -------- | ------ | ---- | -------------------------------- |
| dialogId | number | 是 | openCustomDialog返回的对话框id。 |
**错误码:**
以下错误码的详细介绍请参见[通用错误码](../errorcode-universal.md)和[ohos.promptAction(弹窗)](errorcode-promptAction.md)错误码。
| 错误码ID | 错误信息 |
| -------- | ---------------------------------- |
| 401 | Parameter error. Possible causes: 1. Mandatory parameters are left unspecified; 2.Incorrect parameters types; 3. Parameter verification failed. |
| 100001 | Internal error. |
**示例:**
示例请看[promptAction.openCustomDialog](#promptactionopencustomdialog11)的示例。
## ShowToastOptions
文本提示框的选项。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 类型 | 必填 | 说明 |
| ----------------------- | ------------------------------------------------------------ | ---- | ------------------------------------------------------------ |
| message | string \| [Resource](arkui-ts/ts-types.md#resource类型) | 是 | 显示的文本信息。
**说明:**
默认字体为'Harmony Sans',不支持设置其他字体。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| duration | number | 否 | 默认值1500ms,取值区间:1500ms-10000ms。若小于1500ms则取默认值,若大于10000ms则取上限值10000ms。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| bottom | string \| number | 否 | 设置弹窗底部边框距离导航条的高度,ToastShowMode.TOP_MOST模式下,软键盘拉起时,如果bottom值过小,toast要被软键盘遮挡时,会自动避让至距离软键盘80vp处。ToastShowMode.DEFAULT模式下,软键盘拉起时,会上移软键盘的高度。
默认值:80vp
**说明:**
当底部没有导航条时,bottom为设置弹窗底部边框距离窗口底部的高度。
设置对齐方式alignment后,bottom不生效。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| showMode11+ | [ToastShowMode](#toastshowmode11) | 否 | 设置弹窗是否显示在应用之上。
默认值:ToastShowMode.DEFAULT,默认显示在应用内。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| alignment12+ | [Alignment](arkui-ts/ts-appendix-enums.md#alignment) | 否 | 对齐方式。
默认值:undefined,默认底部偏上位置。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| offset12+ | [Offset](arkui-ts/ts-types.md#offset) | 否 | 在对齐方式上的偏移。
默认值:{ dx: 0, dy: 0 },默认没有偏移。
**说明:**
只支持设置px类型的数值,如需设置vp,可以将vp改成px传入。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| backgroundColor12+ | [ResourceColor](arkui-ts/ts-types.md#resourcecolor) | 否 | 文本提示框背板颜色。
默认值:Color.Transparent
**说明:**
当设置了backgroundColor为非透明色时,backgroundBlurStyle需要设置为BlurStyle.NONE,否则颜色显示将不符合预期效果。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| textColor12+ | [ResourceColor](arkui-ts/ts-types.md#resourcecolor) | 否 | 文本提示框文本颜色。
默认值:Color.Black
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| backgroundBlurStyle12+ | [BlurStyle](arkui-ts/ts-universal-attributes-background.md#blurstyle9) | 否 | 文本提示框背板模糊材质。
默认值:BlurStyle.COMPONENT_ULTRA_THICK
**说明:**
设置为BlurStyle.NONE即可关闭背景虚化。当设置了backgroundBlurStyle为非NONE值时,则不要设置backgroundColor,否则颜色显示将不符合预期效果。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| shadow12+ | [ShadowOptions](arkui-ts/ts-universal-attributes-image-effect.md#shadowoptions对象说明) \| [ShadowStyle](arkui-ts/ts-universal-attributes-image-effect.md#shadowstyle10枚举说明) | 否 | 文本提示框背板阴影。
默认值:ShadowStyle.OUTER_DEFAULT_MD
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| enableHoverMode14+ | boolean | 否 | 是否响应悬停态。
默认值:False,默认不响应。
**原子化服务API:** 从API version 14开始,该接口支持在原子化服务中使用。 |
| hoverModeArea14+ | [HoverModeAreaType](arkui-ts/ts-appendix-enums.md#hovermodeareatype14) | 否 | 响应悬停态时,弹窗的显示区域。
默认值:HoverModeAreaType.BOTTOM_SCREEN,默认显示在下半屏。
**原子化服务API:** 从API version 14开始,该接口支持在原子化服务中使用。 |
## ToastShowMode11+
设置弹窗显示模式,默认显示在应用内,支持显示在应用之上。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 值 | 说明 |
| -------- | ---- | ---------------------- |
| DEFAULT | 0 | Toast 显示在应用内。 |
| TOP_MOST | 1 | Toast 显示在应用之上。 |
## ShowDialogOptions
对话框的选项。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 类型 | 必填 | 说明 |
| --------------------------------- | ------------------------------------------------------------ | ---- | ------------------------------------------------------------ |
| title | string \| [Resource](arkui-ts/ts-types.md#resource类型) | 否 | 标题文本。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| message | string \| [Resource](arkui-ts/ts-types.md#resource类型) | 否 | 内容文本。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| buttons | Array<[Button](#button)> | 否 | 对话框中按钮的数组,结构为:{text:'button', color: '\#666666'},支持大于1个按钮。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| alignment10+ | [DialogAlignment](arkui-ts/ts-methods-alert-dialog-box.md#dialogalignment枚举说明) | 否 | 弹窗在竖直方向上的对齐方式。
默认值:DialogAlignment.Default
**说明**:
若在UIExtension中设置showInSubWindow为true, 弹窗将基于UIExtension的宿主窗口对齐。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| offset10+ | [Offset](arkui-ts/ts-types.md#offset) | 否 | 弹窗相对alignment所在位置的偏移量。
默认值:{ dx: 0 , dy: 0 }
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| maskRect10+ | [Rectangle](arkui-ts/ts-methods-alert-dialog-box.md#rectangle8类型说明) | 否 | 弹窗遮蔽层区域,在遮蔽层区域内的事件不透传,在遮蔽层区域外的事件透传。
默认值:{ x: 0, y: 0, width: '100%', height: '100%' }
**说明:**
showInSubWindow为true时,maskRect不生效。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| showInSubWindow11+ | boolean | 否 | 某弹框需要显示在主窗口之外时,是否在子窗口显示此弹窗。
默认值:false,弹窗显示在应用内,而非独立子窗口。
**说明**:showInSubWindow为true的弹窗无法触发显示另一个showInSubWindow为true的弹窗。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| isModal11+ | boolean | 否 | 弹窗是否为模态窗口,模态窗口有蒙层,非模态窗口无蒙层。
默认值:true,此时弹窗有蒙层。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| backgroundColor12+ | [ResourceColor](arkui-ts/ts-types.md#resourcecolor) | 否 | 弹窗背板颜色。
默认值:Color.Transparent
**说明:**
当设置了backgroundColor为非透明色时,backgroundBlurStyle需要设置为BlurStyle.NONE,否则颜色显示将不符合预期效果。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| backgroundBlurStyle12+ | [BlurStyle](arkui-ts/ts-universal-attributes-background.md#blurstyle9) | 否 | 弹窗背板模糊材质。
默认值:BlurStyle.COMPONENT_ULTRA_THICK
**说明:**
设置为BlurStyle.NONE即可关闭背景虚化。当设置了backgroundBlurStyle为非NONE值时,则不要设置backgroundColor,否则颜色显示将不符合预期效果。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| shadow12+ | [ShadowOptions](arkui-ts/ts-universal-attributes-image-effect.md#shadowoptions对象说明) \| [ShadowStyle](arkui-ts/ts-universal-attributes-image-effect.md#shadowstyle10枚举说明) | 否 | 设置弹窗背板的阴影。
当设备为2in1时,默认场景下获焦阴影值为ShadowStyle.OUTER_FLOATING_MD,失焦为ShadowStyle.OUTER_FLOATING_SM
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| enableHoverMode14+ | boolean | 否 | 是否响应悬停态。
默认值:false,默认不响应。
**原子化服务API:** 从API version 14开始,该接口支持在原子化服务中使用。 |
| hoverModeArea14+ | [HoverModeAreaType](arkui-ts/ts-appendix-enums.md#hovermodeareatype14) | 否 | 悬停态下弹窗默认展示区域。
默认值:HoverModeAreaType.BOTTOM_SCREEN
**原子化服务API:** 从API version 14开始,该接口支持在原子化服务中使用。 |
## ShowDialogSuccessResponse
对话框的响应结果。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 类型 | 必填 | 说明 |
| ----- | ------ | ---- | ------------------------------- |
| index | number | 是 | 选中按钮在buttons数组中的索引。 |
## ActionMenuOptions
操作菜单的选项。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 类型 | 必填 | 说明 |
| ----------------------------- | ------------------------------------------------------------ | ---- | ------------------------------------------------------------ |
| title | string \| [Resource](arkui-ts/ts-types.md#resource类型) | 否 | 标题文本。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| buttons | [[Button](#button),[Button](#button)?,[Button](#button)?,[Button](#button)?,[Button](#button)?,[Button](#button)?] | 是 | 菜单中菜单项按钮的数组,结构为:{text:'button', color: '\#666666'},支持1-6个按钮。按钮数量大于6个时,仅显示前6个按钮,之后的按钮不显示。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| showInSubWindow11+ | boolean | 否 | 某弹框需要显示在主窗口之外时,是否在子窗口显示此弹窗。
默认值:false,在子窗口不显示弹窗。
**说明**:
- showInSubWindow为true的弹窗无法触发显示另一个showInSubWindow为true的弹窗。
- 若在UIExtension中设置showInSubWindow为true, 弹窗将基于UIExtension的宿主窗口对齐。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| isModal11+ | boolean | 否 | 弹窗是否为模态窗口,模态窗口有蒙层,非模态窗口无蒙层。
默认值:true,此时弹窗有蒙层。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
## ActionMenuSuccessResponse
操作菜单的响应结果。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 类型 | 必填 | 说明 |
| ----- | ------ | ---- | ---------------------------------------- |
| index | number | 是 | 选中按钮在buttons数组中的索引,从0开始。 |
## CustomDialogOptions11+
自定义弹窗的内容,继承自[BaseDialogOptions](#basedialogoptions11)。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 类型 | 必填 | 说明 |
| ------- | ------------------------------------------------------- | ---- | ------------------------------------------------------------ |
| builder | [CustomBuilder](arkui-ts/ts-types.md#custombuilder8) | 是 | 设置自定义弹窗的内容。
**说明:**
builder需要赋值为箭头函数,格式如下:() => { this.XXX() },其中XXX是内部builder名。
如果是全局builder需要在组件内部创建一个builder,在内部builder中调用全局builder。
builder根节点宽高百分比相对弹框容器大小。
builder非根节点宽高百分比相对父节点大小。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| backgroundColor 12+| [ResourceColor](arkui-ts/ts-types.md#resourcecolor) | 否 | 设置弹窗背板颜色。
默认值:Color.Transparent
**说明:**
当设置了backgroundColor为非透明色时,backgroundBlurStyle需要设置为BlurStyle.NONE,否则颜色显示将不符合预期效果。 |
| cornerRadius12+| [Dimension](arkui-ts/ts-types.md#dimension10) \| [BorderRadiuses](arkui-ts/ts-types.md#borderradiuses9) | 否 | 设置背板的圆角半径。
可分别设置4个圆角的半径。
默认值:{ topLeft: '32vp', topRight: '32vp', bottomLeft: '32vp', bottomRight: '32vp' }
圆角大小受组件尺寸限制,最大值为组件宽或高的一半,若值为负,则按照默认值处理。
百分比参数方式:以父元素弹窗宽和高的百分比来设置弹窗的圆角。|
| borderWidth12+| [Dimension](arkui-ts/ts-types.md#dimension10) \| [EdgeWidths](arkui-ts/ts-types.md#edgewidths9) | 否 | 设置弹窗背板的边框宽度。
可分别设置4个边框宽度。
默认值:0
百分比参数方式:以父元素弹窗宽的百分比来设置弹窗的边框宽度。
当弹窗左边框和右边框大于弹窗宽度,弹窗上边框和下边框大于弹窗高度,显示可能不符合预期。 |
| borderColor12+ | [ResourceColor](arkui-ts/ts-types.md#resourcecolor) \| [EdgeColors](arkui-ts/ts-types.md#edgecolors9) | 否 | 设置弹窗背板的边框颜色。
默认值:Color.Black
如果使用borderColor属性,需要和borderWidth属性一起使用。 |
| borderStyle12+ | [BorderStyle](arkui-ts/ts-appendix-enums.md#borderstyle) \| [EdgeStyles](arkui-ts/ts-types.md#edgestyles9) | 否 | 设置弹窗背板的边框样式。
默认值:BorderStyle.Solid
如果使用borderStyle属性,需要和borderWidth属性一起使用。 |
| width12+ | [Dimension](arkui-ts/ts-types.md#dimension10) | 否 | 设置弹窗背板的宽度。
**说明:**
- 弹窗宽度默认最大值:400vp。
- 百分比参数方式:弹窗参考宽度为所在窗口的宽度,在此基础上调小或调大。|
| height12+ | [Dimension](arkui-ts/ts-types.md#dimension10) | 否 | 设置弹窗背板的高度。
**说明:**
- 弹窗高度默认最大值:0.9 *(窗口高度 - 安全区域)。
- 百分比参数方式:弹窗参考高度为(窗口高度 - 安全区域),在此基础上调小或调大。|
| shadow12+| [ShadowOptions](arkui-ts/ts-universal-attributes-image-effect.md#shadowoptions对象说明) \| [ShadowStyle](arkui-ts/ts-universal-attributes-image-effect.md#shadowstyle10枚举说明) | 否 | 设置弹窗背板的阴影。
当设备为2in1时,默认场景下获焦阴影值为ShadowStyle.OUTER_FLOATING_MD,失焦为ShadowStyle.OUTER_FLOATING_SM |
| backgroundBlurStyle12+ | [BlurStyle](arkui-ts/ts-universal-attributes-background.md#blurstyle9) | 否 | 弹窗背板模糊材质。
默认值:BlurStyle.COMPONENT_ULTRA_THICK
**说明:**
设置为BlurStyle.NONE即可关闭背景虚化。当设置了backgroundBlurStyle为非NONE值时,则不要设置backgroundColor,否则颜色显示将不符合预期效果。 |
## BaseDialogOptions11+
弹窗的选项。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 类型 | 必填 | 说明 |
| --------------- | ------------------------------------------------------------ | ---- | ------------------------------------------------------------ |
| maskRect | [Rectangle](arkui-ts/ts-methods-alert-dialog-box.md#rectangle8类型说明) | 否 | 弹窗遮蔽层区域。
默认值:{ x: 0, y: 0, width: '100%', height: '100%' }
**说明:**
showInSubWindow为true时,maskRect不生效。 |
| alignment | [DialogAlignment](arkui-ts/ts-methods-alert-dialog-box.md#dialogalignment枚举说明) | 否 | 弹窗在竖直方向上的对齐方式。
默认值:DialogAlignment.Default
**说明**:
若在UIExtension中设置showInSubWindow为true, 弹窗将基于UIExtension的宿主窗口对齐。|
| offset | [Offset](arkui-ts/ts-types.md#offset) | 否 | 弹窗相对alignment所在位置的偏移量。
默认值:{ dx: 0 , dy: 0 } |
| isModal | boolean | 否 | 弹窗是否为模态窗口,模态窗口有蒙层,非模态窗口无蒙层。
默认值:true,此时弹窗有蒙层。 |
| showInSubWindow | boolean | 否 | 某弹框需要显示在主窗口之外时,是否在子窗口显示此弹窗。
默认值:false,弹窗显示在应用内,而非独立子窗口。 |
| onWillDismiss12+ | Callback<[DismissDialogAction](#dismissdialogaction12)> | 否 | 交互式关闭回调函数。
**说明:**
1.当用户执行点击遮障层关闭、左滑/右滑、三键back、键盘ESC关闭交互操作时,如果注册该回调函数,则不会立刻关闭弹窗。在回调函数中可以通过reason得到阻拦关闭弹窗的操作类型,从而根据原因选择是否能关闭弹窗。当前组件返回的reason中,暂不支持CLOSE_BUTTON的枚举值。
2.在onWillDismiss回调中,不能再做onWillDismiss拦截。 |
| autoCancel12+ | boolean | 否 | 点击遮障层时,是否关闭弹窗,true表示关闭弹窗。false表示不关闭弹窗。
默认值:true |
| maskColor12+ | [ResourceColor](arkui-ts/ts-types.md#resourcecolor) | 否 | 自定义蒙层颜色。
默认值: 0x33000000 |
| transition12+ | [TransitionEffect](arkui-ts/ts-transition-animation-component.md#transitioneffect10) | 否 | 设置弹窗显示和退出的过渡效果。
**说明:**
1.如果不设置,则使用默认的显示/退出动效。
2.显示动效中按back键,打断显示动效,执行退出动效,动画效果为显示动效与退出动效的曲线叠加后的效果。
3.退出动效中按back键,不会打断退出动效,退出动效继续执行,继续按back键退出应用。 |
| onDidAppear12+ | () => void | 否 | 弹窗弹出时的事件回调。
**说明:**
1.正常时序依次为:onWillAppear>>onDidAppear>>(onDateAccept/onCancel/onDateChange)>>onWillDisappear>>onDidDisappear。
2.在onDidAppear内设置改变弹窗显示效果的回调事件,二次弹出生效。
3.快速点击弹出,消失弹窗时,存在onWillDisappear在onDidAppear前生效。
4. 当弹窗入场动效未完成时关闭弹窗,该回调不会触发。 |
| onDidDisappear12+ | () => void | 否 | 弹窗消失时的事件回调。
**说明:**
正常时序依次为:onWillAppear>>onDidAppear>>(onDateAccept/onCancel/onDateChange)>>onWillDisappear>>onDidDisappear。 |
| onWillAppear12+ | () => void | 否 | 弹窗显示动效前的事件回调。
**说明:**
1.正常时序依次为:onWillAppear>>onDidAppear>>(onDateAccept/onCancel/onDateChange)>>onWillDisappear>>onDidDisappear。
2.在onWillAppear内设置改变弹窗显示效果的回调事件,二次弹出生效。 |
| onWillDisappear12+ | () => void | 否 | 弹窗退出动效前的事件回调。
**说明:**
1.正常时序依次为:onWillAppear>>onDidAppear>>(onDateAccept/onCancel/onDateChange)>>onWillDisappear>>onDidDisappear。
2.快速点击弹出,消失弹窗时,存在onWillDisappear在onDidAppear前生效。 |
| keyboardAvoidMode12+ | [KeyboardAvoidMode](#keyboardavoidmode12枚举说明) | 否 | 用于设置弹窗是否在拉起软键盘时进行自动避让。
默认值:KeyboardAvoidMode.DEFAULT
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |
| enableHoverMode14+ | boolean | 否 | 是否响应悬停态。
默认值:false,默认不响应。
**原子化服务API:** 从API version 14开始,该接口支持在原子化服务中使用。|
| hoverModeArea14+ | [HoverModeAreaType](arkui-ts/ts-appendix-enums.md#hovermodeareatype14) | 否 | 悬停态下弹窗默认展示区域。
默认值:HoverModeAreaType.BOTTOM_SCREEN
**原子化服务API:** 从API version 14开始,该接口支持在原子化服务中使用。|
## DismissDialogAction12+
Dialog关闭的信息。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
### 属性
| 名称 | 类型 | 可读 | 可写 | 说明 |
| ------- | ------------------------------------------------------------ | ---- | ---- | ------------------------------------------------------------ |
| dismiss | Callback<void> | 否 | 否 | Dialog关闭回调函数。开发者需要退出时调用,不需要退出时无需调用。 |
| reason | [DismissReason](#dismissreason12枚举说明) | 否 | 否 | Dialog无法关闭原因。根据开发者需要选择不同操作下,Dialog是否需要关闭。 |
## DismissReason12+枚举说明
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 值 | 描述 |
| ------------- | ---- | ------------------------------------------------------------ |
| PRESS_BACK | 0 | 点击三键back、左滑/右滑、键盘ESC。 |
| TOUCH_OUTSIDE | 1 | 点击遮障层时。 |
| CLOSE_BUTTON | 2 | 点击关闭按钮。 |
| SLIDE_DOWN | 3 | 下拉关闭。
**说明:**
该接口仅支持在[半模态转场](./arkui-ts/ts-universal-attributes-sheet-transition.md)中使用。 |
## KeyboardAvoidMode12+枚举说明
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 值 | 说明 |
| ------- | ---- | ------------------------------------------------ |
| DEFAULT | 0 | 默认避让软键盘并在到达极限高度之后进行高度压缩。 |
| NONE | 1 | 不避让软键盘。 |
## Button
菜单中的菜单项按钮。
**系统能力:** SystemCapability.ArkUI.ArkUI.Full
| 名称 | 类型 | 必填 | 说明 |
| ----- | ---------------------------------------- | ---- | ------- |
| text | string \| [Resource](arkui-ts/ts-types.md#resource类型) | 是 | 按钮文本内容。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| color | string \| [Resource](arkui-ts/ts-types.md#resource类型) | 是 | 按钮文本颜色。
**原子化服务API:** 从API version 11开始,该接口支持在原子化服务中使用。 |
| primary12+ | boolean | 否 | 在弹窗获焦且未进行tab键走焦时,按钮是否默认响应Enter键。多个Button时,只允许一个Button的该字段配置为true,否则所有Button均不响应。多重弹窗可自动获焦连续响应。
**原子化服务API:** 从API version 12开始,该接口支持在原子化服务中使用。 |